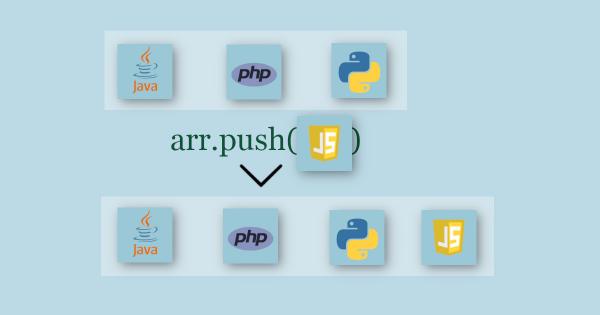
Javascript has a number of methods related to arrays that allow programmers to perform various array operations. There are four methods that are particularly used for adding and removing elements to and from an array. They are: push()
, pop()
, shift()
and unshift()
. For experienced as well as new programmers, its likely to sometimes get confused about how each of them work and which one to use in which situation. Thus, in this article, we have tried to simplify the concept with pictures and examples. Let's start exploring them one by one. Then we will compare their similarities and differences. Please look at the pictures too for better understanding.
1. Push
As seen in the image above, the push()
the method adds one or more elements at the end of an array. Those element(s) are supplied as parameters while calling the method. This phenomenon of putting things at the end of something(a file, an array, etc.) is often called 'append' in the computer world. After appending the elements(s), push()
the method returns the new length of the array.
// Syntax: arr.push(item1[, ...[, itemN]])
// Parameters: One or more items
// Return value: the new length of array
let languages = [ "Java", "PHP", "Python" ];
let foo = languages.push("JS");
console.log(foo);
// Output => 4
console.log(languages);
// Output => [ 'Java', 'PHP', 'Python', 'JS' ]
2. Pop
The pop()
method removes the last element from an array and returns that element. This method does not take any parameter.
// Syntax: arr.pop()
// Return value: the removed element of the array
let languages = ["Java", "PHP", "Python"];
let poppedItem = languages.pop();
console.log(poppedItem);
// Output => Python
console.log(languages);
// Output => [ 'Java', 'PHP' ]
3. Shift
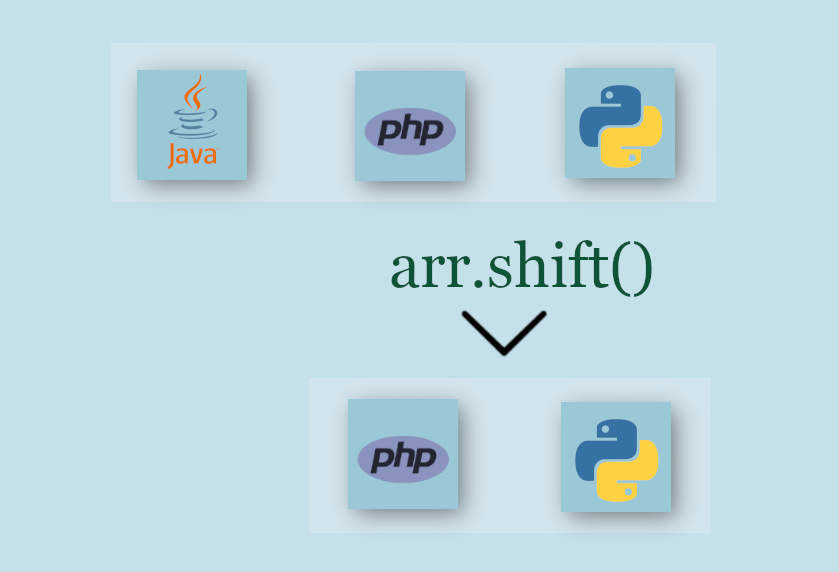
The shift()
method is similar to the pop()
method. It removes the first element from an array and returns it. Just like pop()
method, it does not take any parameter.
// Syntax: arr.shift()
// Return value: the removed element of the array
let languages = ["Java", "PHP", "Python"];
let foo = languages.shift();
console.log(foo);
// Output => Java
console.log(languages);
// Output => [ 'PHP', 'Python' ]
4. Unshift
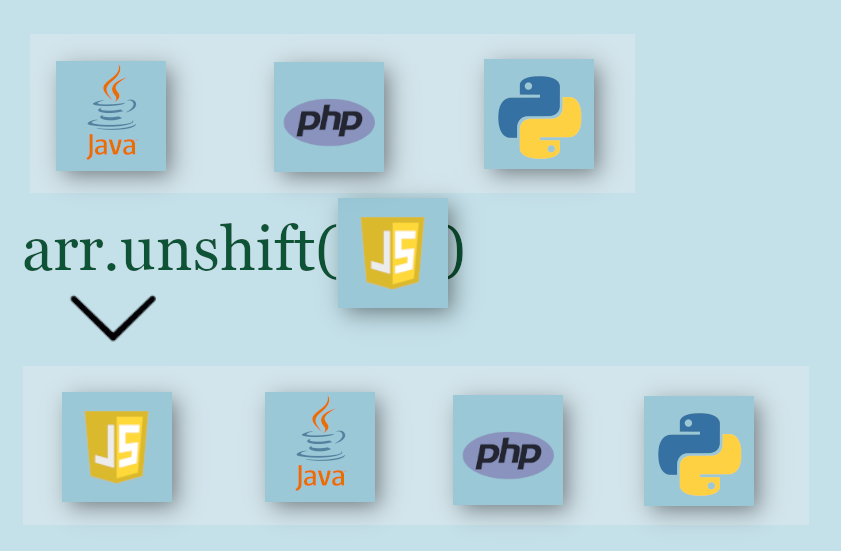
Just like the push()
method we saw in the beginning, unshift()
adds one or more elements to the array. But it adds them at the beginning of the array, which is often called as prepend in computer science. After prepending the element(s), the unshift()
method returns the new length value of the array.
//Syntax: arr.unshift(Item1[, ...[, ItemN]])
// Return value: the new length of the array
let languages = ["Java", "PHP", "Python"];
let foo = languages.unshift("JS");
console.log(foo);
// Output => 4
console.log(languages);
// Output => [ 'JS', 'Java', 'PHP', 'Python' ]
By now, I am hopeful that your concepts are clear. Let us use the comparison method now which will help us summarize it understand it even better. Comparing will also help us retain the concept for a longer time.
'Push and Pop' vs 'Shift and Unshift'
Push
and Pop
deal with the end of the array while Shift
and Unshift
deal with the beginning of the array.
Push vs Pop
Push
is for adding element(s) while Pop
is for removing an element.
Push
requires elements as parameters when being invoked while Pop
does not need the same.
Push
returns the new length of the array while Pop
returns the popped out element.
Unshift vs Shift
Unshift
is for adding element(s) while Shift
is for removing an element.
Unshift
requires elements as parameters when being invoked while Shift
does not need the same.
Unshift
returns the new length of the array while Shift
returns the removed element.
I hope it helped. Let's discuss more on the comments section?
If you liked this article, I recommend another one related to javascript's slice()
method. It has graphics to help you understand the slice() methods, negative indexing and concept of shallow copy.